Salesforce Batch Apex Interview Questions and Answers
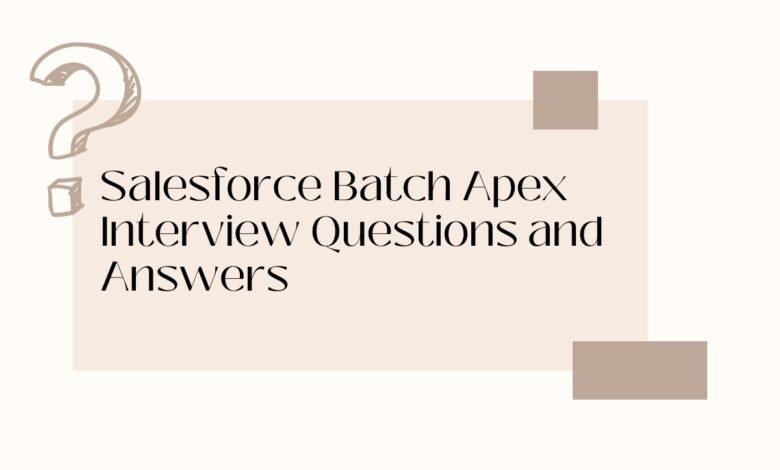
1. What is Batch Apex in Salesforce?
Batch Apex allows you to handle more records than a typical Apex job. It processes data in batches to stay within platform limits. You can use Batch Apex to build complex, long-running processes on the Salesforce platform.
2. How does Batch Apex differ from regular Apex?
Batch Apex is specifically designed for processing large data sets that exceed the governor limits when processed all at once. Unlike regular Apex, which is executed in one go, Batch Apex divides the workload into smaller batches, which are processed sequentially.
3. Can you explain the basic structure of a Batch Apex class?
A Batch Apex class implements the Salesforce-provided ‘Database.Batchable’ interface. It consists of three methods: ‘start’, ‘execute’, and ‘finish’. The ‘start’ method is used to collect the records or objects to be processed. ‘execute’ processes each batch of records, and ‘finish’ runs once after all batches are processed.
public class batch implements Database.Batchable <sObject> { public (Database.QueryLocator or Iterable<sobject>) start(Database.BatchableContext bc) { //query on object; //return Database.getQueryLocator(query); } public void execute(Database.batchableContext bc, List <SObject> scope) { //some processing } public void finish(Database.BatchableContext bc) { //job such as sending email or calling another batch class } }
4. How do you schedule Batch Apex for execution?
To schedule Batch Apex, you can implement the Schedulable interface and use the System.schedule
method.
This method returns the scheduled job ID also called CronTrigger ID.
String cronID = System.scheduleBatch(reassign, 'job example', 1);
CronTrigger ct = [SELECT Id, TimesTriggered, NextFireTime
FROM CronTrigger WHERE Id = :cronID];
5. What are Governor Limits in Batch Apex, and how do you handle them?
Governor Limits in Salesforce are runtime limits enforced to ensure the efficient use of resources. In Batch Apex, to manage these limits, operations are broken down into smaller batches, each executed separately to prevent limit exceptions.
6. Describe how you would use the Stateful interface in Batch Apex.
The Stateful interface allows you to maintain state across different batches. When you implement this interface, instance variables retain their values between batches. It’s useful for counting or summing records processed or holding a reference to data that you use throughout the execution of the batch job.
7. How does the Batch Apex ‘execute’ method work?
The ‘execute’ method is invoked by Salesforce once for each batch of records processed. It contains the code responsible for performing operations on each record in the batch. The method receives two parameters: a reference to the Database.BatchableContext and a collection of records to process.
8. What is chaining in Batch Apex and how is it used?
Chaining allows you to link multiple batch jobs to run one after the other. This is achieved by starting a new batch job from the ‘finish’ method of a current batch job. Chaining is used when subsequent batch processes depend on the completion of the previous one.
9. Can you call future method from Batch Apex?
No, you cannot directly call future method from within a batch class. Instead, Batch Apex should be used as it provides better flexibility in processing large numbers of asynchronous operations.
10. What happens if a batch job fails during execution?
If a batch job fails, Salesforce executes the ‘finish’ method, if provided, where you can implement error handling logic. Additionally, Salesforce sends an email to the Apex job’s submitter with error details. It’s also recommended to have a mechanism to retry or to
11. How do you monitor and manage Batch Apex jobs in Salesforce?
Batch Apex jobs can be monitored in the Salesforce UI using the Apex Jobs page or programmatically using the AsyncApexJob
object. Management involves monitoring job statuses, rescheduling jobs if necessary, and handling failed jobs effectively.
The Database.executeBatch
method returns the ID of the AsyncApexJob
object.
ID batchprocessid = Database.executeBatch(reassign); AsyncApexJob aaj = [SELECT Id, Status, JobItemsProcessed, TotalJobItems, NumberOfErrors FROM AsyncApexJob WHERE ID =: batchprocessid ];
12. What is Database.QueryLocator & Iterable<sObject>?
In Database.QueryLocator, we use a simple query (SELECT) to generate the scope of objects. The governor limit for the total number of records retrieved by SOQL queries is bypassed, i.e. it can return up to 50 million records.
In Iterable<sObject>, we can create a custom scope for processing, which would be not possible to create using SOQL where clauses. the governor limit for the total number of records retrieved by SOQL queries is still enforced.
13. If We use Itereable<sObject>, will We be able to process Batches in a specific order?
Batches of records tend to execute in the order in which they’re received from the start method. However, the order in which batches of records execute depends on various factors. The order of execution isn’t guaranteed. So even if you do ORDER BY, there is no guarantee of processing in the same order.
14. Can I query related records using Database.QueryLocator?
Yes, You can do subquery for related records, but with a relationship subquery, the batch job processing becomes slower. A better strategy is to perform the subquery separately, from within the execute method, which allows the batch job to run using the faster.
15. Can I Use FOR UPDATE in SOQL using Database.QueryLocator?
No, We can’t. It will through an exception stating that “Locking is implied for each batch execution and therefore FOR UPDATE should not be specified”
The reason is, If we query the entire database of an org using SELECT … FOR UPDATE, we would lock the entire database as long as the batch was active.
16. Let’s say Record A has to be processed before Record B, but Record B came in the first Batch and Record A came in the second batch. The batch picks records which are unprocessed every time it runs. How will you control the processing Order?
The Processing order can’t be controlled, but we can bypass the record B processing before Record A. We can implement Database.STATEFUL and use one class variable to track whether Record A has processed or not. If not processed and Record B has come, don’t process Record B. After all the execution completes, Record A has already been processed so Run the batch again, to process Record B.
17. Is there any other interface that can be implemented by a Batch apex?
Database.AllowsCallouts is another Marker Interface, which is implemented to make HTTP callouts through Batch.
18. In what scenario might you use Database.AllowsCallouts with Batch Apex?
You would implement Database.AllowsCallouts
in situations where you need to make external web service callouts during the batch process. This interface allows batch classes to make HTTP callouts after performing DML operations.
19. What is the Limit of Size of scope in a batch?
If the start method of the batch class returns a QueryLocator, the optional scope parameter of Database.executeBatch can have a maximum value of 2,000. If set to a higher value, Salesforce chunks the records returned by the QueryLocator into smaller batches of up to 2,000 records. If the start method of the batch class returns an iterable, the scope parameter value has no upper limit.
20. Let’s say, we have run an apex batch to process 1000 records, and It is running with batch size 200. Now, while doing DML on 398th record, an error occurred, What will happen in that case?
In batches, If the first transaction succeeds but the second fails, the database updates made in the first transaction are not rolled back.
Since the batch size is 200, so the first batch will be processed completely and all data will be committed to DB. In seconds batch, if we are committing records using normal DML statements like insert, update than the whole batch will be rollbacked. So records 201 to 400 will not be processed.
If we use the Database DML operations like Database.insert with AllOrNone as false, then partial commit can happen and only 398th record will not be processed in that batch and total 199 records will be processed. Also, the other batch execution will not gonna be hampered.
21. How can you stop a Batch job?
The Database.executeBatch and System.scheduleBatch method returns an ID that can be used in System.abortJob method.
22. Let’s say, I have 150 Batch jobs to execute, Will I be able to queue them in one go?
Once you run Database.executeBatch, the Batch jobs will be placed in the Apex flex queue and its status becomes Holding. The Apex flex queue has the maximum number of 100 jobs, Database.executeBatch throws a LimitException and doesn’t add the job to the queue. So atmost 100 jobs can be added in one go.
Also, if Apex flex queue is not enabled, the Job status becomes Queued, Since the concurrent limit of the queued or active batch is 5, so atmost 5 batch jobs can be added in one go.
23. Can I change the order of already queued Batch Jobs?
Only jobs having status as Holding can be moved. It can be done through UI of Apex Flex queue or we can use Apex Flex Queue Methods.
Boolean isSuccess = System.FlexQueue.moveBeforeJob(jobToMoveId, jobInQueueId);
24. How Can I Test a Batch Apex?
We can test it by calling the Batch job between Test.startTest() and Test.stopTest(). Using the Test methods startTest and stopTest around the executeBatch method to ensure that it finishes before continuing your test. All asynchronous calls made after the startTest method are collected by the system. When stopTest is executed, all asynchronous processes are run synchronously.
Also we can test only one execution of the execute method. So we need to set the scope as per the governer limits.
25. What strategies can you employ to ensure Batch Apex jobs run efficiently?
To ensure efficiency, optimize your query in the start
method to retrieve only necessary records, respect governor limits when setting your batch size, write efficient code in the execute
method, and handle exceptions properly.