Salesforce Queueable Apex Interview Questions
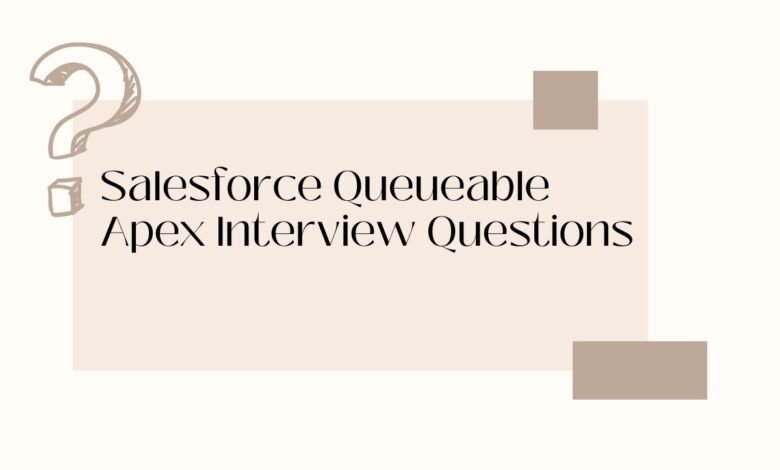
1. What is Queueable Apex?
Queueable Apex is a powerful feature in Salesforce that allows you to execute long-running processes asynchronously. Think of it as a way to offload heavy tasks, like extensive database operations or external web service callouts, to the background so they don’t impact the performance of your application. Queueable apex can be called from the Future and Batch class.
Here’s how Queueable Apex differs from future method:
- Chaining Jobs: One standout feature of Queueable Apex is the ability to chain one job to another. This means you can set up a sequence of tasks to execute in a specific order, which is incredibly useful for complex processing workflows.
- Job Identification: When you submit a job using Queueable Apex, you receive an ID for that job. This allows you to track and monitor the progress of your asynchronous processes more effectively.
- Handling Non-Primitive Types: Unlike @future methods, Queueable Apex lets you work with non-primitive data types, such as sObjects or custom Apex types. This means you can pass more complex data structures between your synchronous and asynchronous code.
By leveraging Queueable Apex, you can improve the scalability and performance of your Salesforce applications while also taking advantage of advanced features like job chaining and handling non-primitive types.
2. Which interface is implemented for Queueable class?
Queueable class implements Queueable
3. What are methods used in Queueable Apex Class?
Execute method
4. Write a sample Queueable Job?
To implement Queueable Apex, you can create a class that implements the Queueable interface and overrides the execute method. Here’s an example:
public class QueueableApexExample implements Queueable { public void execute(QueueableContext context) { // Your processing logic goes here // For example, querying records, performing calculations, or making callouts } }
5. How many jobs can you chain from executing a job?
You can add only one job from an executing job, which means that only one child job can exist for each parent job
6. How many jobs can you queue in a single transaction?
You can add up to 50 jobs to the queue with System.enqueueJob in a single transaction.
7. How can I use Job Id to trace the Job?
Just perform a SOQL query on AsyncApexJob by filtering on the job ID.
AsyncApexJob jobInfo = [SELECT Status,NumberOfErrors FROM AsyncApexJob WHERE Id=:jobID];
8. Can I do callouts from a Queueable Job?
Yes, you have to implement the Database.AllowsCallouts interface to do callouts from Queueable Jobs.
9. If I have written more than one System.enqueueJob call, what will happen?
System will throw LimitException stating “Too many queueable jobs added to the queue: N”
10. How to call more than one Queueable Jobs from a Batch apex, how can I achieve it?
Since we can’t call more than one Queueable Job from each execution Context, We can go for scheduling the Queueable Jobs.
The approach is we need to first check how many queueable jobs are added in the queue in the current transaction by making use of Limits class. If the number has reached the limit, then call a schedulable class and enqueue the queueable class from the execute method of a schedulable class.
11. What is QueueableContext?
It is an interface that is implemented internally by Apex, and contains the job ID. Once you queue the Queueable Job, the Job Id will be returned to you, by apex through QueueableContext’s getJobId() method.
12. How can I queue above Job(QueueableApexExample)?
Using System.enqueueJob Method.
ID jobID = System.enqueueJob(new QueueableApexExample());
13. I have 200 records to be processed using Queueable Apex, How Can I divide the execution Context for every 100 records?
Similar to future jobs, queueable jobs don’t process batches, so you can’t divide the execution Context. It will process all 200 records, in a single execution Context.
14. Can I do callouts from a Queueable Job?
Yes, you have to implement the Database.AllowsCallouts interface to do callouts from Queueable Jobs.
15. How Chaining works in Queueable Apex?
Chaining allows us to customize the execution order of a set of jobs in a process.
let’s say there are N jobs, A, B, C and so on. We have a process in which A has to run first and gives output to B and then B runs and gives output to C and so on. So, instead of calling the individual Queueable Jobs explicitly, we can link the calls, logically within Queueable Jobs, as shown Below
public class A implements Queueable { public void execute(QueueableContext context) { // Chain this job to next job by submitting the next job System.enqueueJob(new B()); } }public class B implements Queueable { public void execute(QueueableContext context) { // Chain this job to next job by submitting the next job System.enqueueJob(new C()); } }public class C implements Queueable { public void execute(QueueableContext context) { // Chain this job to next job by submitting the next job System.enqueueJob(new D()); } }and so on...
17. Can I call Queueable from a batch?
Yes, But you’re limited to just one System.enqueueJob call per execute in the Database.Batchable class. Salesforce has imposed this limitation to prevent explosive execution.
18. How can we handle this error, without using a try-catch?
We can check whether the current count of queuable jobs has exceeded the limit or not, if not, queue them.
Limits.getQueueableJobs() returns the currently queued jobs count.
Limits.getLimitQueueableJobs() returns the limit.
If(Limits.getQueueableJobs() < Limits.getLimitQueueableJobs()) { //System.enqueueJob() }
20. how to test a Queueable Job?
A queueable job is an asynchronous process. To ensure that this process runs within the test method, the job is submitted to the queue between the Test.startTest and Test.stopTest block.
Also, The ID of a queueable Apex job isn’t returned in test context — System.enqueueJob returns null in a running test.
21. how to test Chaining?
You can’t chain queueable jobs in an Apex test. So you have to write separate test cases for each chained queueable job. Also, while chaining the jobs, add a check of Test.isRunningTest() before calling the enqueueJob.
public class A implements Queueable {
public void execute(QueueableContext context) {
// Chain this job to next job by submitting the next job and it's context should not be a test case.
if(!Test.isRunningTest()){
System.enqueueJob(new B());
}
}
}