Salesforce Future Methods Interview Questions
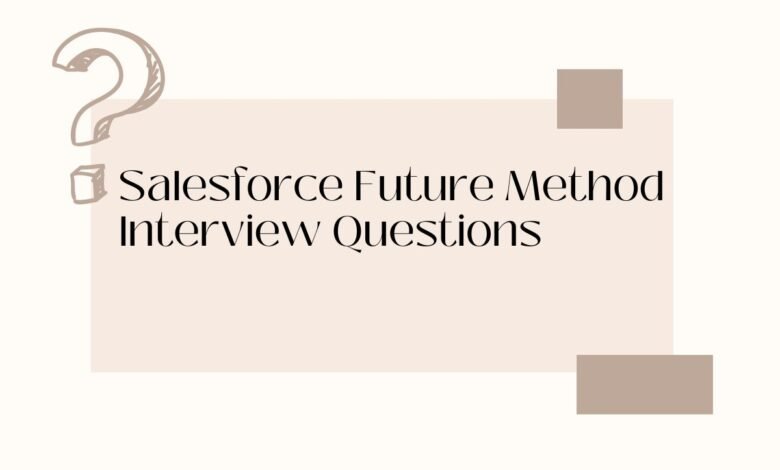
1. What is Future Methods?
Future Methods in Salesforce allow developers to offload non-urgent tasks to be executed asynchronously, separate from the main execution flow. By annotating a method with the @future annotation, developers can instruct Salesforce to process it in the background, without delaying the user’s interaction with the application.
Method with the future annotation must be static method, and can only return a void type.
public class FutureClass { @future public static void myFutureMethod(){ // Perform some operations } }
2. Why do we use Future Method?
You can call a future method for executing long-running operations, such as callouts to external Web services or any operation you’d like to run in its own thread, on its own time.
3. What kinds of parameters supported in Future method?
You can pass primitive data types, arrays of primitive data types, or collections of primitive data types as parameters. No sObjects OR objects as arguments can be passed.
4. Why sobject type parameters are not supported in Future method?
The reason why sObjects can’t be passed as arguments to future method is that the sObject might change between the time you call the method and the time it executes. In this case, the future method will get the old sObject values and might overwrite them.
5. What could be the workaround for sobject types?
To work with sObjects, pass the sObject ID instead (or collection of IDs) and use the ID to perform a query for the most up-to-date record.
public class FutureMethodRecordProcessing
{
@future
public static void processRecords(List<ID> recordIds)
{
// Get those records based on the IDs
// Process records
}
}
6. How can I perform Callouts from Future method?
We need to add a parameter callout=true in @future.
public class FutureMethodExample
{
@future(callout=true)
public static void doCallouts(String name)
{
// Perform a callout to an external service
}
}
7. How Can I call a future method?
You can invoke future methods the same way you invoke any other method.
FutureMethodExample.doCallouts('Account');
8. Can I Write the Above statement in a Batch Job?
No you can’t, because Calling a future method is not allowed in the Batch Jobs.
9. Can I write a future call in Trigger?
Yes, you can.
10. So, consider a case, I have Written a future call in the Account’s trigger update operation. and I have a batch job running on Account records and does DML on them. Will the future call be invoked after DML?
Since you are in batch context, the trigger runs in the same context too, So as soon as the Account records get updated through the batch process or through any future method, the trigger would throw an exception saying “Future method cannot be called from a future or batch method” as a future method cannot be invoked from future or batch method execution context.
11. How can avoid this Exception condition, Without using try-catch?
We can update the trigger logic to leverage the System.isFuture() and System.isBatch() calls so that the future method invocation is not made if the current execution context is future or batch.
trigger AccountTrigger on Account (after insert, after update) { if(!System.isFuture() && !System.isBatch()){ // future call } }
12. So In Any case, I can’t call a future method from a batch Job?
Calling a future method is not allowed in the Execute method, But a web service can be called. A web service can also call an @future method. So, we can define a web service having a future method invocation and call the web service from the execute method of Batch Job.
13. How Many Future methods can be defined in a Class?
Any number of. There are no restrictions as such.
14. What are the other use cases of using a future method?
We can also use future methods to isolate DML operations on different sObject types to prevent the mixed DML error.
15. How Future method helps in avoiding Mixed DML errors?
We can shift DML operations of a particular kind in the Future scope. Since both the DML operations are isolated from each other, the transaction doesn’t fail.
16. Once I call a future method, How can I trace its execution?
Salesforce uses a queue-based framework to handle asynchronous processes from such sources as future methods and batch Apex. So, We can check the apex jobs if it has run or not.
But if it in the queue, and resources are not available, It won’t show up on Apex jobs Page, So we can poll AsyncApexJob object to get its status.
However, future methods don’t return an ID, so We can’t trace it directly. We can use another filter such as MethodName, or JobType, to find the required job.
17. How to test a future method?
To test methods defined with the future annotation, call the class containing the method in a startTest(), stopTest() code block. All asynchronous calls made after the startTest method are collected by the system. When stopTest is executed, all asynchronous processes are run synchronously.
18. What are some limitations of future method?
Some of the limitations are
- It is not a good option to process large numbers of records.
- Only primitive data types supported.
- Tracing a future job is also typical.
- Can’t call future from batch and future contexts, 1 call from queueable context is allowed.
19. Can we fix the order in which future method will run?
Future methods are not guaranteed to execute in the same order as they are called. When using future methods, it’s also possible that two future methods could run concurrently, which could result in record locking.
20. How to call Future methods from flow?
To call Future methods from flow, call the future method from the invocable method.
21. Can we pass wrapper to future method?
You can pass wrapper, but for that, you’ll need to serialize/deserialize that parameter. You can convert the Wrapper to a String which is a primitive.
Once converted into a String you can them pass that string as a parameter to the future method in consideration.
22. Can I call a future method from another future method?
No, you cannot call one future method from another future method.
23. What is the limit on future calls per apex invocation?
50, additionally there are limits base on the 24-hour time frame.
24. Why does the future method not support sObject data types as arguments?
The reason why sObject can’t be passed as arguments to future methods is because the sObject might change between the time we call the method and the time it executes. In this case ,the future method will get the old sObject values and might overwrite them.